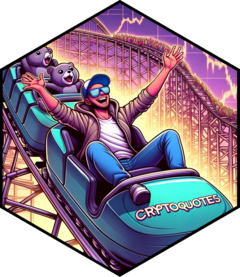
Cryptocurrency Market Data in R
The cryptoQuotes way
Source:vignettes/articles/00-introduction.Rmd
00-introduction.Rmd
## load library
library(cryptoQuotes)
The main goal of the cryptoQuotes
-package is to bridge
the gap between R
and the cryptocurrency market data. Its a
high-level API
-client that connects to major cryptocurrency
exchanges and their respective public market data endpoints.
In this article we will focus on price
and
sentiment
data made available by the Kraken exchange.
Cryptocurrency market data
In this section we will focus on market data from the last 24 hours, on the hourly chart.
OHLC data
To get OHLC
data the get_quote()
-function
is the go-to function,
## Get the
## SPOT price of
## Bitcoin on the hourly
BTC <- get_quote(
ticker = "BTCUSD",
source = "kraken",
futures = FALSE,
interval = "1h",
from = Sys.Date() - 1
)
Index | open | high | low | close | volume |
---|---|---|---|---|---|
2024-05-18 06:00:00 | 66881.5 | 66946.4 | 66820 | 66946.4 | 7.863 |
2024-05-18 07:00:00 | 66946.4 | 67207.7 | 66908.2 | 66927 | 109.901 |
2024-05-18 08:00:00 | 66927.1 | 67066.6 | 66927 | 67028.2 | 13.307 |
2024-05-18 09:00:00 | 67028.2 | 67137 | 67028.1 | 67137 | 7.185 |
2024-05-18 10:00:00 | 67137 | 67283 | 67042.4 | 67234.1 | 57.78 |
2024-05-18 11:00:00 | 67234.1 | 67362.9 | 67191 | 67200.1 | 20.063 |
Sentiment data
One sentiment indicator for Bitcoin is the long-short ratio, which
can be retrieved using get_lsratio()
-function,
## Get the
## long-short ratio of
## Bitcoin on the hourly
LS_BTC <- get_lsratio(
ticker = "PF_XBTUSD",
source = "kraken",
interval = "1h",
from = Sys.Date() - 1
)
Index | long | short | ls_ratio |
---|---|---|---|
2024-05-18 06:00:00 | 0.715 | 0.285 | 2.514 |
2024-05-18 07:00:00 | 0.71 | 0.29 | 2.442 |
2024-05-18 08:00:00 | 0.708 | 0.292 | 2.429 |
2024-05-18 09:00:00 | 0.712 | 0.288 | 2.469 |
2024-05-18 10:00:00 | 0.713 | 0.287 | 2.487 |
2024-05-18 11:00:00 | 0.707 | 0.293 | 2.411 |
Limitations
There is a limit to the amount of market data that can be extracted
in one call. The Kraken exchange,
for example, has a limit on 5000
rows of data per call in
the futures market,
## Get the SPOT
## market for over
## 2000 rows
tryCatch(
get_quote(
ticker = "PF_XBTUSD",
source = "kraken",
futures = TRUE,
interval = "5m",
from = Sys.Date() - 25,
to = Sys.Date()
),
error = function(error) {
error
}
)
#> <error/rlang_error>
#> Error in `base::tryCatch()`:
#> ✖ Time period too large, too many bars (7200), maximum is 5000!
#> ---
#> Backtrace:
#> ▆
#> 1. ├─base::tryCatch(...)
#> 2. │ └─base (local) tryCatchList(expr, classes, parentenv, handlers)
#> 3. │ └─base (local) tryCatchOne(expr, names, parentenv, handlers[[1L]])
#> 4. │ └─base (local) doTryCatch(return(expr), name, parentenv, handler)
#> 5. └─cryptoQuotes::get_quote(...)
#> 6. └─cryptoQuotes:::fetch(...)
#> 7. ├─cryptoQuotes:::flatten(...)
#> 8. └─cryptoQuotes:::GET(...)
#> 9. └─base::tryCatch(...)
#> 10. └─base (local) tryCatchList(expr, classes, parentenv, handlers)
#> 11. └─base (local) tryCatchOne(expr, names, parentenv, handlers[[1L]])
#> 12. └─value[[3L]](cond)
If you need more data than this, you need to do multiple calls. One such solution is the following,
## 1) create date
## sequence
dates <- seq(
from = as.POSIXct(Sys.Date()),
by = "-5 mins",
length.out = 10000
)
## 2) split the sequence
## in multiples of 100
## by assigning numbers
## to each indices of 100
idx <- rep(
x = 1:2,
each = 5000
)
## 3) use the idx to split
## the dates into equal parts
split_dates <- split(
x = dates,
f = idx
)
## 4) collect all all
## calls in a list
## using lapply
ohlc <- lapply(
X = split_dates,
FUN = function(dates){
Sys.sleep(1)
cryptoQuotes::get_quote(
ticker = "PF_XBTUSD",
source = "kraken",
futures = TRUE,
interval = "5m",
from = min(dates),
to = max(dates)
)
})
## 4.1) rbind all
## elements
nrow(
ohlc <- do.call(
what = rbind,
args = ohlc
)
)
#> [1] 10000
Note: For an indepth analysis of the various limitations and workarounds please see the
cryptoQuotes
wiki on Github